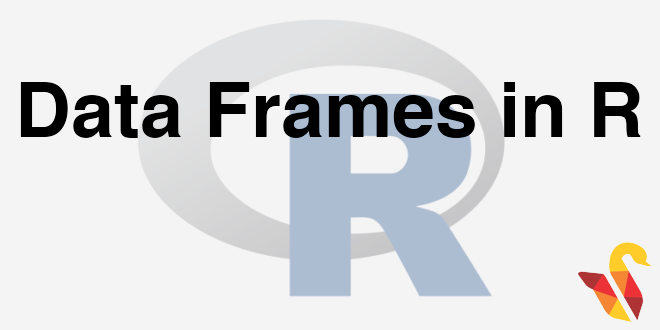
In previous section, we studied about R Data Types, now we will be studying about R Data Frames.
A data frame is a common way for storing data in R. We can create data frames using function data.frame
Here are four vectors of the same length,
>Name<-c("Rick","Nick","Harry") >Age<-c(15,17,19) >English<-c(12,13,20) >Maths<-c(20,23,21)
A data frame, students has been created using the individual vectors Name, Age, English, and Maths.
>students<-data.frame(Name,Age,English,Maths) >students Name Age English Maths 1 Rick 15 12 20 2 Nick 17 13 23 3 Harry 19 20 21
>Profile_data <- data.frame(Name, Age) >Profile_data
Name Age 1 Rick 15 2 Nick 17 3 Harry 19
>students1 <-c(Name, Age, English, Maths)
>students1
[1] "Rick" "Nick" "Harry" "15" "17" "19" "12" "13" "20" "20" "23"
[12] "21"
Accessing a Data Frame
To access each column of a data frame, we use ‘$’. We write it as- Dataset$column
students$Name [1] Rick Nick Harry Levels: Harry Nick Rick
We have other ways-
>students$English [1] 12 13 20
>students["Name"] Name 1 Rick 2 Nick 3 Harry >students[1,] Name Age English Maths 1 Rick 15 12 20
>students[,1] [1] Rick Nick Harry Levels: Harry Nick Rick
>students[,2:4] Age English Maths 1 15 12 20 2 17 13 23 3 19 20 21
>students[,-1] Age English Maths 1 15 12 20 2 17 13 23 3 19 20 21
>students[-1,] Name Age English Maths 2 Nick 17 13 23 3 Harry 19 20 21
>students[,c(1,4)] Name Maths 1 Rick 20 2 Nick 23 3 Harry 21
There is a slight Difference in Accessed Data frame elements in these commands.
>students$Name [1] Rick Nick Harry Levels: Harry Nick Rick
>students["Name"] Name 1 Rick 2 Nick 3 Harry
>students[,1] [1] Rick Nick Harry Levels: Harry Nick Rick
Built-in DataFrames
When we install R, then R comes with built-in Datasets. These are some of the useful open source datasets for practice. We can call them by command: data()
data() #Air Passengers data AirPassengers
Jan Feb Mar Apr May Jun Jul Aug Sep Oct Nov Dec ## 1949 112 118 132 129 121 135 148 148 136 119 104 118 ## 1950 115 126 141 135 125 149 170 170 158 133 114 140 ## 1951 145 150 178 163 172 178 199 199 184 162 146 166 ## 1952 171 180 193 181 183 218 230 242 209 191 172 194 ## 1953 196 196 236 235 229 243 264 272 237 211 180 201 ## 1954 204 188 235 227 234 264 302 293 259 229 203 229 ## 1955 242 233 267 269 270 315 364 347 312 274 237 278 ## 1956 284 277 317 313 318 374 413 405 355 306 271 306 ## 1957 315 301 356 348 355 422 465 467 404 347 305 336 ## 1958 340 318 362 348 363 435 491 505 404 359 310 337 ## 1959 360 342 406 396 420 472 548 559 463 407 362 405 ## 1960 417 391 419 461 472 535 622 606 508 461 390 432
Cars data set is included with car speed and stopping distance.
#cars data cars
## speed dist ## 1 4 2 ## 2 4 10 ## 3 7 4 ## 4 7 22 ## 5 8 16 ## 6 9 10 ## 7 10 18 ## 8 10 26 ## 9 10 34 ## 10 11 17 ## 11 11 28 ## 12 12 14 ## 13 12 20 ## 14 12 24 ## 15 12 28 ## 16 13 26 ## 17 13 34 ## 18 13 34 ## 19 13 46 ## 20 14 26 ## 21 14 36 ## 22 14 60 ## 23 14 80 ## 24 15 20 ## 25 15 26 ## 26 15 54 ## 27 16 32 ## 28 16 40 ## 29 17 32 ## 30 17 40 ## 31 17 50 ## 32 18 42 ## 33 18 56 ## 34 18 76 ## 35 18 84 ## 36 19 36 ## 37 19 46 ## 38 19 68 ## 39 20 32 ## 40 20 48 ## 41 20 52 ## 42 20 56 ## 43 20 64 ## 44 22 66 ## 45 23 54 ## 46 24 70 ## 47 24 92 ## 48 24 93 ## 49 24 120 ## 50 25 85
This is about Data Frames. Next you will learn about the R Lists.
In next section, we will be studying about R Lists.