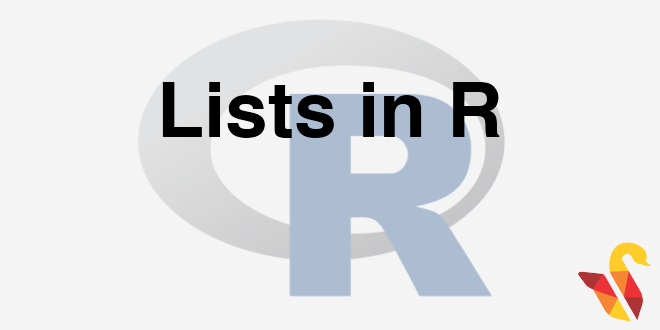
In previous section, we studied about R Data Frames, now we will be studying about R Lists.
R list is a collection of Homogeneous / heterogeneous R components ,i.e., a dataset, a string, an image, an object can be put together. Imagine R objects which should have an image, a dataset, string, etc., then the list will be very useful. Let us see a demo.
x<- c(1:20) //Integer y<-FALSE //Logical z<-"Mike" //String k<-30 l<-attitude Disc<-"This is a list of all my R elements"
Lets look at the datatype of each of these objects
> str(x) int [1:20] 1 2 3 4 5 6 7 8 9 10 ...
> str(y) logi FALSE
> str(z) chr "Mike"
> str(k) num 30
> str(l)
‘data.frame’: 30 obs. of 7 variables:
$ rating : num 43 63 71 61 81 43 58 71 72 67 …
$ complaints: num 51 64 70 63 78 55 67 75 82 61 …
$ privileges: num 30 51 68 45 56 49 42 50 72 45 …
$ learning : num 39 54 69 47 66 44 56 55 67 47 …
$ raises : num 61 63 76 54 71 54 66 70 71 62 …
$ critical : num 92 73 86 84 83 49 68 66 83 80 …
$ advance : num 45 47 48 35 47 34 35 41 31 41 …
We can create a list using list() funcion
> mylist<-list(Disc,x,y,z,k,l) > mylist [[1]] [1] "This is a list of all my R elements" [[2]] [1] 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 [[3]] [1] FALSE [[4]] [1] "Mike" [[5]] [1] 30 [[6]] rating complaints privileges learning raises critical advance 1 43 51 30 39 61 92 45 2 63 64 51 54 63 73 47 3 71 70 68 69 76 86 48 4 61 63 45 47 54 84 35 5 81 78 56 66 71 83 47 6 43 55 49 44 54 49 34 7 58 67 42 56 66 68 35 8 71 75 50 55 70 66 41 9 72 82 72 67 71 83 31 10 67 61 45 47 62 80 41 11 64 53 53 58 58 67 34 12 67 60 47 39 59 74 41 13 69 62 57 42 55 63 25 14 68 83 83 45 59 77 35 15 77 77 54 72 79 77 46 16 81 90 50 72 60 54 36 17 74 85 64 69 79 79 63 18 65 60 65 75 55 80 60 19 65 70 46 57 75 85 46 20 50 58 68 54 64 78 52 21 50 40 33 34 43 64 33 22 64 61 52 62 66 80 41 23 53 66 52 50 63 80 37 24 40 37 42 58 50 57 49 25 63 54 42 48 66 75 33 26 66 77 66 63 88 76 72 27 78 75 58 74 80 78 49 28 48 57 44 45 51 83 38 29 85 85 71 71 77 74 55 30 82 82 39 59 64 78 39
Accessing a list
> mylist[1] [[1]] [1] "This is a list of all my R elements" > mylist[2] [[1]] [1] 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
> mylist[[2]][1] [1] 1
> mylist[6] [[1]] rating complaints privileges learning raises critical advance 1 43 51 30 39 61 92 45 2 63 64 51 54 63 73 47 3 71 70 68 69 76 86 48 4 61 63 45 47 54 84 35 5 81 78 56 66 71 83 47 6 43 55 49 44 54 49 34 7 58 67 42 56 66 68 35 8 71 75 50 55 70 66 41 9 72 82 72 67 71 83 31 10 67 61 45 47 62 80 41 11 64 53 53 58 58 67 34 12 67 60 47 39 59 74 41 13 69 62 57 42 55 63 25 14 68 83 83 45 59 77 35 15 77 77 54 72 79 77 46 16 81 90 50 72 60 54 36 17 74 85 64 69 79 79 63 18 65 60 65 75 55 80 60 19 65 70 46 57 75 85 46 20 50 58 68 54 64 78 52 21 50 40 33 34 43 64 33 22 64 61 52 62 66 80 41 23 53 66 52 50 63 80 37 24 40 37 42 58 50 57 49 25 63 54 42 48 66 75 33 26 66 77 66 63 88 76 72 27 78 75 58 74 80 78 49 28 48 57 44 45 51 83 38 29 85 85 71 71 77 74 55 30 82 82 39 59 64 78 39
The sixth element of our list is dataframe.
mylist[[6]][1]#Note the double braces [[]] in this command
## rating ## 1 43 ## 2 63 ## 3 71 ## 4 61 ## 5 81 ## 6 43 ## 7 58 ## 8 71 ## 9 72 ## 10 67 ## 11 64 ## 12 67 ## 13 69 ## 14 68 ## 15 77 ## 16 81 ## 17 74 ## 18 65 ## 19 65 ## 20 50 ## 21 50 ## 22 64 ## 23 53 ## 24 40 ## 25 63 ## 26 66 ## 27 78 ## 28 48 ## 29 85 ## 30 82
> mylist[[6]][[1]][1] ## [1] 43
There is a difference between List and Data frames. We can’t create a data frame with heterogeneous components, but a list can take all heterogeneous R objects and stores them as new List.
Other Datatypes
There are other data types like factors and Matrices. These data types work well with a specific set of problems.
Factor
The factor is a categorical variable. There is already ‘string’ for a non-numeric variable, but Factor is a bit more like if we have categories “East, West, North, South”, rather than storing these as strings, we can use them as Factor and do categorical data analysis. Factor is a good feature in the statistics where categorical data should be handled very carefully.
Matrix
Matrix is a multi-dimensional array. It has its own advantages while computing specific class of problems.
Demo
> gender<-c("Male","Female") > str(gender) chr [1:2] "Male" "Female"
> gender1<-factor(gender) > str(gender1) Factor w/ 2 levels "Female","Male": 2 1 This is the end to the session datatypes in R. In next section, we will be studying about R Functions.