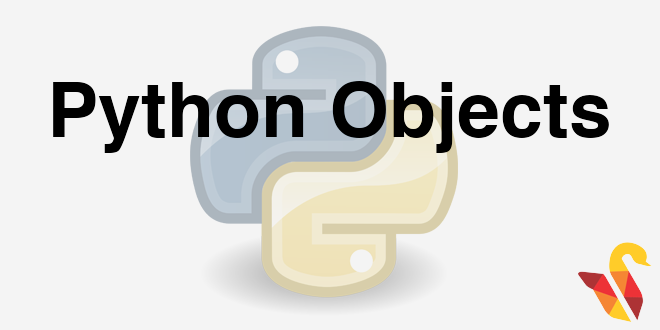
Link to the previous post : https://statinfer.com/104-1-2-python-environment/
In this post we will go through some basic python objects.
Type of Objects
- Object to refer to any entity in a python program.
- Python has some standard built in object types
- Numbers
- Strings
- Lists
- Tuples
- Dictionaries
- Having a good knowledge on these basic objects is essential to fee comfortable in Python programming.
Numbers
- Numbers: integers & floats
In [39]:
age=30
age
Out[39]:
In [34]:
weight=102.88
weight
Out[34]:
In [35]:
x=17
x**2 #Square of x
Out[35]:
Check the variable types for age and weight in variable explorer
In [37]:
type(age)
Out[37]:
In [38]:
type(weight)
Out[38]:
Strings
Strings are amongst the most popular types in Python. There are a number of methods or built-in string functions
Defining Strings
In [40]:
name="Sheldon"
msg="Data Vedi Data Science Classes"
Accessing strings
In [41]:
print(name[0])
print(name[1])
- This is as good as substring
In [42]:
print(msg[0:9])
length of string
In [44]:
len(msg)
Out[44]:
In [43]:
print(msg[10:len(msg)])
Displaying string multiple time
In [45]:
msg="Site under Construction"
msg*10
msg*50
Out[45]:
- There is a difference between print and just displaying a variable.
In [47]:
message="Data Science on R and Data Science on Python \n"
message*10
Out[47]:
In [46]:
print(message*10)
String Concatenation
In [48]:
msg1="Site under Construction "
msg2=msg1+"Go to home page \n"
print(msg2)
print(msg2*10)
List
- List is a hybrid datatype
- A sequence of related data
- Similar to array, but all the elements need not be of same type
- Creating a list
In [50]:
mylist1=['Sheldon','Male', 25]
- Accessing list elements
In [52]:
mylist1[0] #Python indexing starts from 1
Out[52]:
In [53]:
mylist1[1]
Out[53]:
In [54]:
mylist1[2]
Out[54]:
- Appending to a list
In [55]:
mylist2=['L.A','No 173', "CR108877"]
final_list=mylist1+mylist2
final_list
Out[55]:
- Updating list elements
In [56]:
final_list[2]=35
final_list
Out[56]:
- Length of list
In [57]:
len(final_list)
Out[57]:
- Deleting an element in list
In [58]:
del final_list[5]
final_list
Out[58]:
Tuples
- Also sequence data types.
- Crated using parenthesis. Lists were created using square brackets.
- Tuples can’t be updated.
Tuples example:
In [60]:
my_tuple=('Mark','Male', 55)
my_tuple
Out[60]:
In [62]:
my_tuple[1]
Out[62]:
In [63]:
my_tuple[2]
Out[63]:
In [61]:
my_tuple[0]*10
Out[61]:
- Difference between tuples and lists
In [65]:
my_list=['Sheldon','Male', 25]
my_tuple=('Mark','M', 55)
my_list[2]=30
my_list
Out[65]:
In [68]:
my_tuple[2]=40
Dictionaries
- Dictionaries have two major element types key and Value.
- Dictionaries are collection of key value pairs
- Each key is separated from its value by a colon (:), the items are separated by commas, and the whole thing is enclosed in curly braces.
- Keys are unique within a dictionary
In [71]:
city={0:"LA", 1:"PA" , 2:"FL"}
city
Out[71]:
In [72]:
city[0]
Out[72]:
In [73]:
city[1]
Out[73]:
In [70]:
city[2]
Out[70]:
- In dictionary, keys are similar to indexes. We define our own preferred indexes in dictionaries.
- Make sure that we give the right key index while accessing the elements in dictionary.
In [74]:
names={1:"David", 6:"Bill", 9:"Jim"}
names
Out[74]:
In [82]:
names[0] #Doesn't work, because we haven't assign "0" to any value?
In [77]:
names[1]
Out[77]:
In [79]:
names[2]
In [80]:
names[6]
Out[80]:
In [81]:
names[9]
Out[81]:
- In the key value pairs, key doesn’t always need to be a number.
In [83]:
edu={"David":"Bsc", "Bill":"Msc", "Jim":"Phd"}
edu
Out[83]:
In [86]:
edu[0]
In [87]:
edu[1]
In [88]:
edu[David]
In [89]:
edu["David"]
Out[89]:
- Updating values in dictionary
In [90]:
edu
Out[90]:
In [91]:
edu["David"]="MSc"
edu
Out[91]:
- Updating keys in dictionary.
- Delete the key and value element first and then add new element.
In [94]:
city={0:"LA", 1:"PA" , 2:"FL"}
#How to make 6 as "LA"
del city[0]
city
Out[94]:
In [93]:
city[6]="LA"
city
Out[93]:
- Fetch all keys and all values separately
In [96]:
city.keys()
Out[96]:
In [95]:
city.values()
Out[95]:
In [97]:
edu.keys()
Out[97]:
In [98]:
edu.values()
Out[98]:
Next post is on conditional operators in python.
Link to the next post : https://statinfer.com/104-1-4-conditional-operators-in-python/