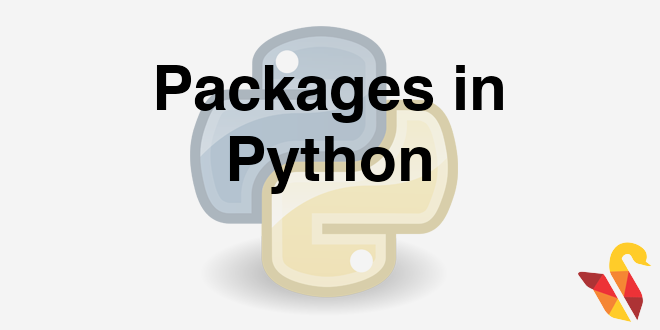
Link to the previous post : https://statinfer.com/104-1-4-conditional-operators-in-python/
Packages
- A package is collection of python functions. A properly structured and complied code. A package may contain many sub packages.
- Many python functions are only available via “packages” that must be imported.
- For example to find value of log(10) we need to first import match package that has the log function in it
In [113]:
log(10)
exp(5)
sqrt(256)
In [115]:
import math
math.log(10)
Out[115]:
In [116]:
math.exp(5)
Out[116]:
In [117]:
math.sqrt(256)
Out[117]:
- To be a good data scientist on python, on need to be very comfortable with below packages
- Numpy
- Scipy
- Pandas
- Scikit-Learn
- Matplotlib
Important Packages- NumPy
- NumPy is for fast operations on vectors and matrices, including mathematical, logical, shape manipulation, sorting, selecting.
- It is the foundation on which all higher level tools for scientific Python are built
In [118]:
import numpy as np
income = np.array([9000, 8500, 9800, 12000, 7900, 6700, 10000])
print(income)
print(income[0])
In [119]:
expenses=income*0.65
print(expenses)
In [120]:
savings=income-expenses
print(savings)
Important Packages- Pandas
- Data frames and data handling
- Pandas has Data structures and operations for manipulating numerical tables and time series.
In [121]:
import pandas as pd
buyer_profile = pd.read_csv('datasets\\Buyers Profiles\\Train_data.csv')
print(buyer_profile)
In [126]:
buyer_profile.Age
Out[126]:
In [122]:
buyer_profile.Gender
Out[122]:
In [124]:
buyer_profile.Age[0]
Out[124]:
In [125]:
buyer_profile.Age[0:10]
Out[125]:
Important Packages- Scikit-Learn
- Machine learning algorithms made easy
In [127]:
import sklearn as sk
import pandas as pd
air_passengers = pd.read_csv('datasets\\AirPassengers\\AirPassengers.csv')
air_passengers
Out[127]:
In [130]:
x=air_passengers['Promotion_Budget']
x=x.reshape(-1,1)
y=air_passengers['Passengers']
y=y.reshape(-1,1)
In [132]:
from sklearn import linear_model
reg = linear_model.LinearRegression()
reg.fit(x, y)
Out[132]:
In [133]:
print('Coefficients: \n', reg.coef_)
Important Packages- Matplotlib¶
Plotting library similar to MATLAB plots
In [3]:
import numpy as np
import matplotlib as mp
import matplotlib.pyplot
#to print the plot in the notebook:
%matplotlib inline
X = np.random.normal(0,1,1000)
Y = np.random.normal(0,1,1000)
mp.pyplot.scatter(X,Y)
Out[3]: