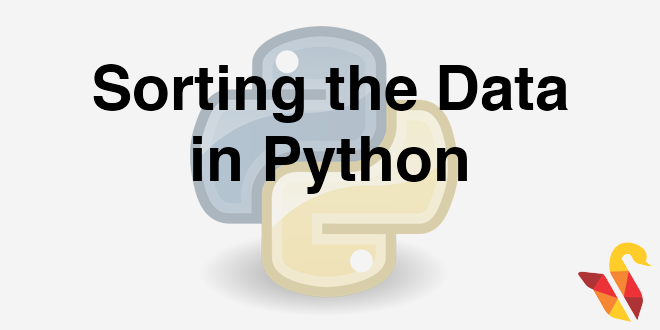
Link to the previous post : https://statinfer.com/104-2-5-subsetting-data-with-variable-filter-condition-in-python/
In the previous post we created subsets of data by condition filtering, in this post we will create the new subsets by sorting one or more column values.
Sorting the data
We will use Online retail dataset.
In [10]:
Online_Retail=pd.read_csv("datasets\\Online Retail Sales Data\\Online Retail.csv", encoding = "ISO-8859-1")
Online_Retail.head(5)
Out[10]:
In [78]:
Online_Retail.columns.values
Out[78]:
- Its ascending by default
In [11]:
Online_Retail_sort=Online_Retail.sort('UnitPrice')
Online_Retail_sort.head(5)
Out[11]:
- we can use
ascending=False
for descending order
In [12]:
Online_Retail_sort=Online_Retail.sort('UnitPrice',ascending=False)
Online_Retail_sort.head(5)
Out[12]:
Practice : Sorting the data
- We will use AutoDataset for this practice which we imported in our previous posts.
- Sort the dataset based on length.
- Sort the dataset based on length descending.
In [83]:
auto_data.columns.values
Out[83]:
In [84]:
auto_data_sort=auto_data.sort( ' length')
auto_data_sort.head(5)
Out[84]:
In [85]:
auto_data_sort1=auto_data.sort( ' length',ascending=False)
auto_data_sort1.head(5)
Out[85]: